How to Dynamically Set the Recipient (To) Email Address in Contact Form 7
Allowing the recipient to be chosen dynamically at page load with Contact Form 7 Dynamic Text Extension adds a lot…
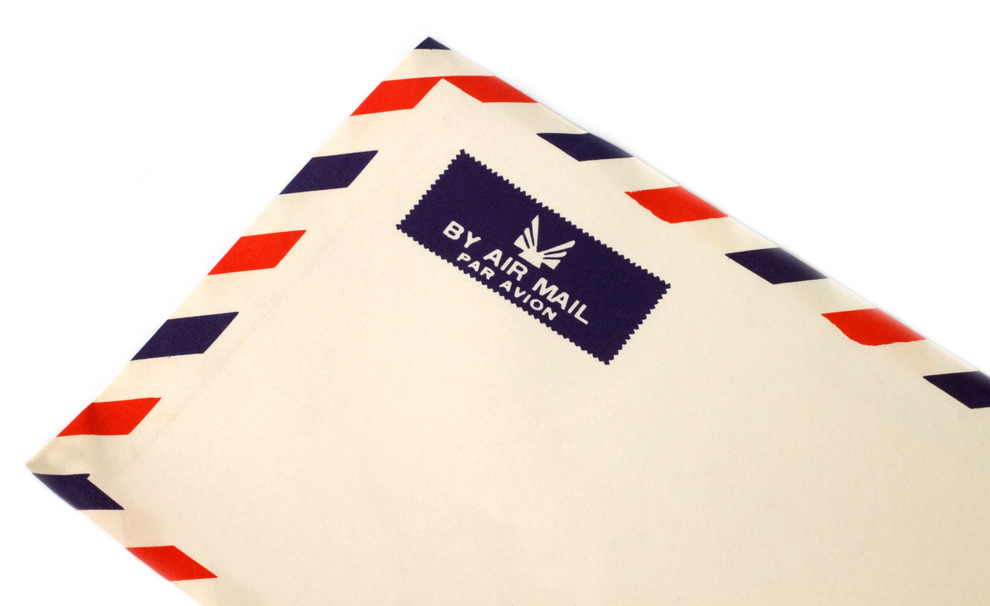
Allowing the recipient to be chosen dynamically at page load with Contact Form 7 Dynamic Text Extension adds a lot…
Here's a sneak peek of my new WordPress mega menu plugin, UberMenu, which will be released in the next few…
Say you want to allow users to contact you, and you want to provide some context for their inquiry. For…
Sorry, comments are closed due to ridiculous amounts of sp*m. Please use the WordPress.org forums for support. Thanks! I've just…
Recently, I had a client who wanted a map of Israel on their site. I started out using Google Maps…
Here's a really cool talk by Steven Johnson on how great ideas come into existence. He describes how ideas form,…